In my summary of iOS adblockers I brought up a topic of using custom DNS servers as a better way of limiting ads and tracking and here's why. On iOS this method allows to limit them even outside Safari, which is very good news, since there are no actually working ways of keeping other apps and services from tracking you unless you jailbreak your device.
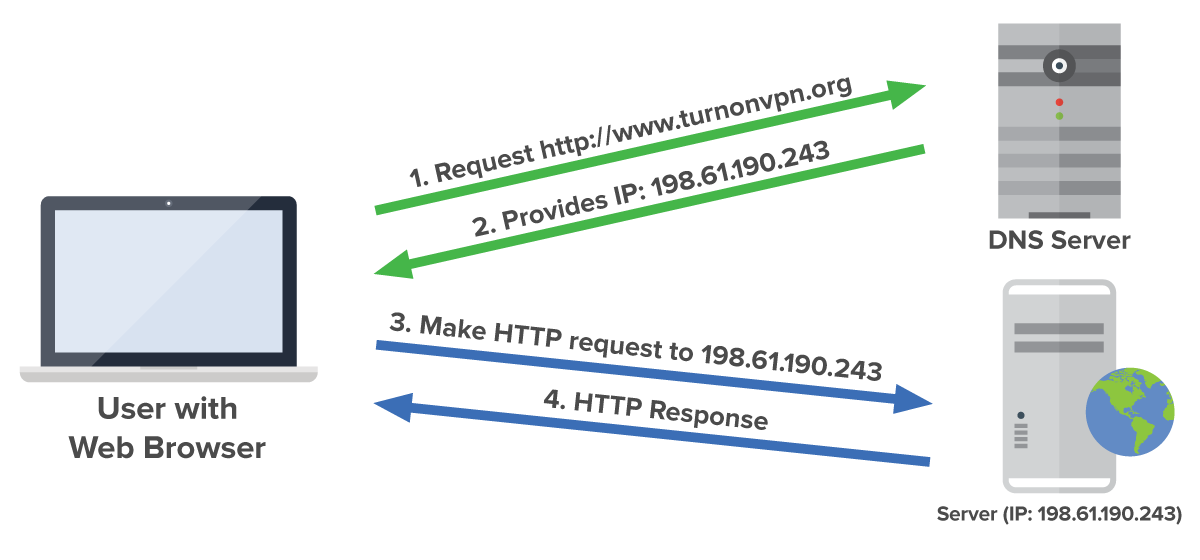
I'll be getting into details of setting custom DNS Servers and DNSCrypt on iOS since it's the most limited platform in terms of options. All of the steps below are available on Windows, Mac and Android as well, which makes it the most versatile option for the most devices possible.
So what is a DNS? DNS is abbreviated from Domain Name System - it's a special network of servers which purpose is just to resolve domains. What that means is that when you enter any address in your browser, or any app or service that you're using connects to its service via a domain, your device which allows that connection connects to its DNS server and asks for the IP address of the domain you're connecting to. If you open google.com, your computer or mobile phone asks the IP address of the domain, receives 216.58.215.78 and connects your browser to it. But if for some reason the DNS server doesn't resolve your DNS request, your browser won't know where to connect and as a result you won't see anything. This is exactly what can be used not to see ads.
DNS is a hierarchical network with few root servers at the top. The system is built to be fail safe with backup servers in each node, but from its nature there were times when a portion of them went down making parts of the Internet inaccessible to some users. For that reason ISPs (Internet service providers) have their own copies of the main DNS servers in case they might temporary fail. They keep those copies up to date with the main servers so when there's an outage the IPSs' users won't feel anything since their DNS queries will still resolve on the ISP servers. Also potentially resolving your DNS queries in place speeds up your loading speeds comparing to the situation when your DNS query would go further than your ISP servers which would just take longer to do.
Usually when you connect your device to any router, it will give you static IP addresses of the DNS servers the router is set up to share. The routers of your ISP are pre-set with their DNS servers, the same happens when your phone gets cellular connection - phone's DNS servers are also set for you. And most ISPs in order to keep users away from messing up with their Internet keep those DNS IP addresses hidden from change.
But why would you want to change your DNS servers? Well there are few reasons for that:
- Your DNS provider (most often your ISP) has his own non-objective interests in mind. If it's a governmental ISP in Russia, they might wanna block their subjectively harmful websites, and not resolving the DNS query for those domains is an easy way of doing it. Your browser would just tell you that it 'couldn't resolve host name'. Or if your ISP provides additional services, they might just block their online competitors that way, leaving you out only with the options they want you to have. These examples are a bit extreme, but worth mentioning since all of the scenarios are easily possible. Even though when blocking, ISPs do a bit further and just block all the traffic to specific resources and not just DNS queries. And in that case only VPNs are your only option to get around the limitations.
- Your DNS provider has less incentive than you on blocking ads and trackers since it sometimes might break the website you're watching or maybe your ISP shareholders are also partial owners of an advertising group, etc. And this is where your custom DNS server might help you out when your ISP won't, by setting up few rules not to resolve the domains which usually serve ads or track your online behavior and identity and afterwards sell that information again to advertisers.
As mentioned before the DNS system consists of root and other big servers that resolve people's queries from all over the world. But not only your ISP has a copy of the domain names and their IP addresses. Any private company or even regular people can set up their own DNS server. The only difference is that the root servers are operating mostly independently, following objective rules and are considered safe to be used by everyone, unlike some Joe's public DNS server. Big companies also have their own DNS servers, in particular Google with its 8.8.8.8 and CloudFare (cloud service provider) with 1.1.1.1. And even though Google is a trusted company, I wouldn't trust them to resolve my DNS queries, even though they probably filter out malicious ones for good reasons, but their core business is about ads, so they may be doing that for competitive reasons, filtering competition out, and leaving only their own domains responsible for tracking 🙂
That's why after CloudFare revealed their DNS few months ago I started using them right away, since their core business relies on making the Internet faster and safer not as a byproduct of serving ads. I couldn't switch my router to serve their DNS to all my home devices since my ISP hid the option, so I had to setup all my devices manually.
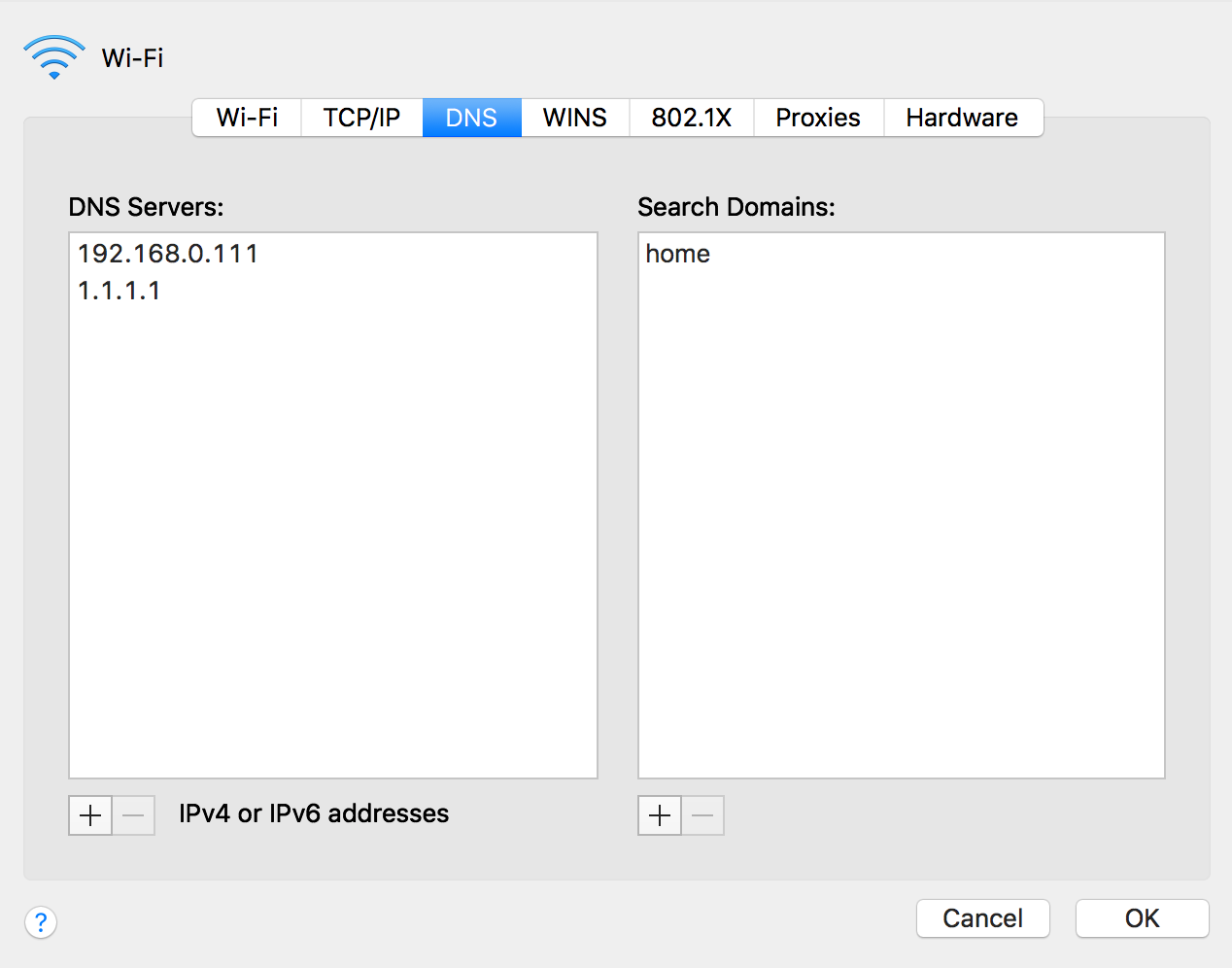
It's very easy doing it on Mac OS: you go to Settings.app -> Network, push 'Advanced' on your connection of choice (Wi-Fi, Ethernet, etc.) and on the 'DNS' tab add 1.1.1.1 and confirm the changes - that easy! You can repeat it on all of your Macs and PCs and this setting will stay even while connecting to other wireless or wired networks, neat!
On Android and iOS the DNS setting is per each wireless network. So if you use few on a constant basis - unfortunately you will have to repeat this process for each of the networks: open Settings.app -> Wi-Fi, tap on the 'i' icon next to your connected network and then on 'Configure DNS' at the bottom, select 'Manual' and enter 1.1.1.1 as well. Save changes, enjoy your faster and more secure Internet 🙂
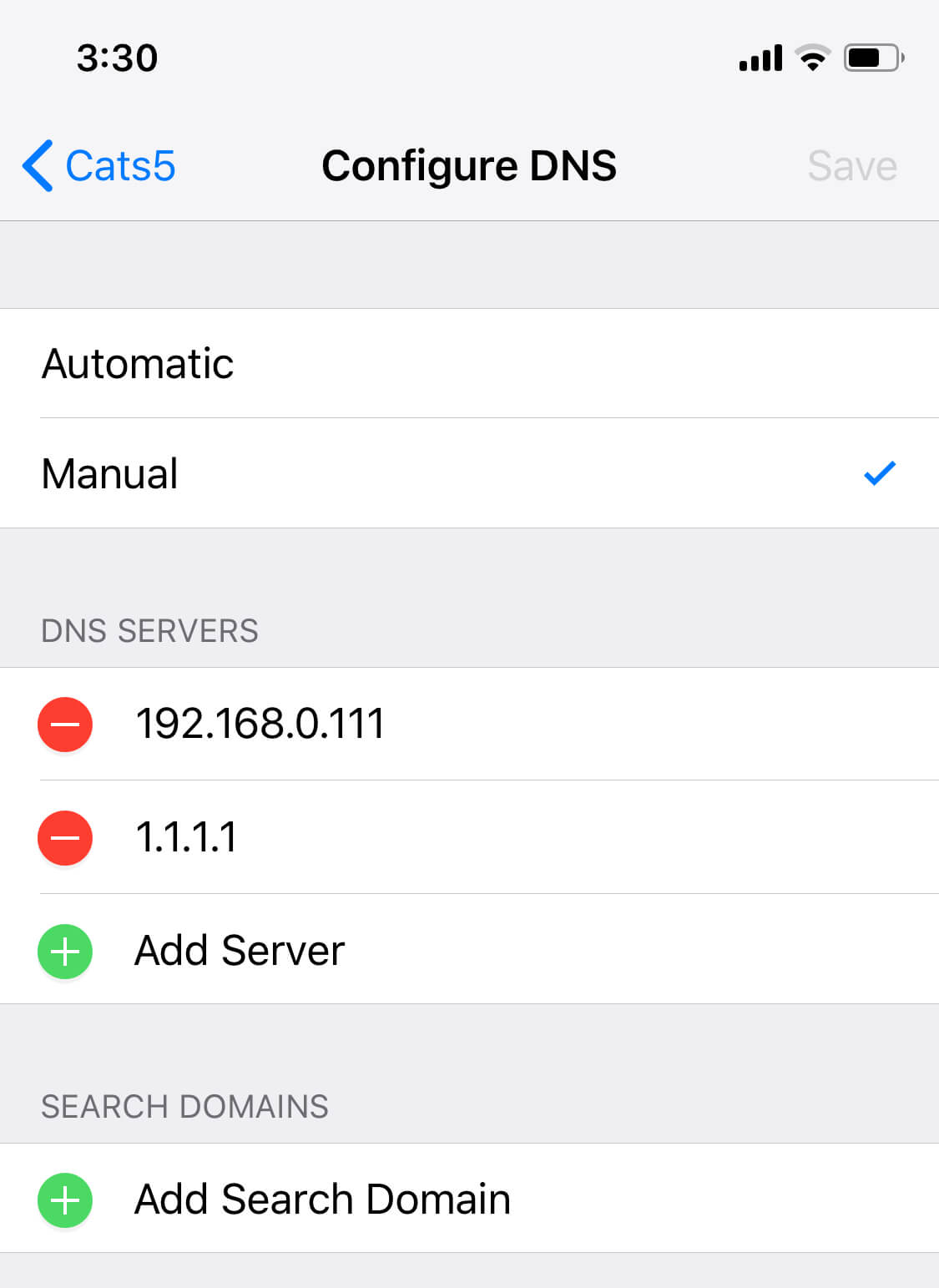
The setup on Android is almost identical and I'm sure you will find it after going through the same sequence.